Adding a real-time unread conversation counter to a TalkJS Chat
Note: This is an old tutorial. TalkJS now has a built-in unread conversations counter!
We’ll show you how to add a real-time unread conversations counter for TalkJS Inbox using the TalkJS Session. We will do this by registering an event handler to listen for unread conversations and render the counter accordingly on a web page.
Setting up a Session
A TalkJS Session represents a user’s active browser tab. The Session is also responsible for authenticating users and your application with TalkJS.
Here, we create a TalkJs user and bind that user to an Inbox instance using the TalkJs Session. Let’s define a TalkJS session using the new Talk.Session(options) constructor and pass our appID and User object.
var user = new Talk.User({
id: "124582", //unique ID for a new user or an existing user's ID
name: "Chris Pratt",
email: "chris@example.com",
photoUrl: "https://i.picsum.photos/id/1025/4951/3301.jpg",
welcomeMessage: "Hey there,welcome to TalkJS!",
role: "default"
});
window.talkSession = new Talk.Session({
appId: "APP_ID", // replace this with your real APP ID
me: user
});
After setting up the Session, it is now time to create a TalkJS inbox so that our user can chat with other TalkJS users.
var inbox = talkSession.createInbox();
// Mount TalkJS Inbox to a Div with id "talkjs-container"
inbox.mount(document.getElementById("talkjs-container"));
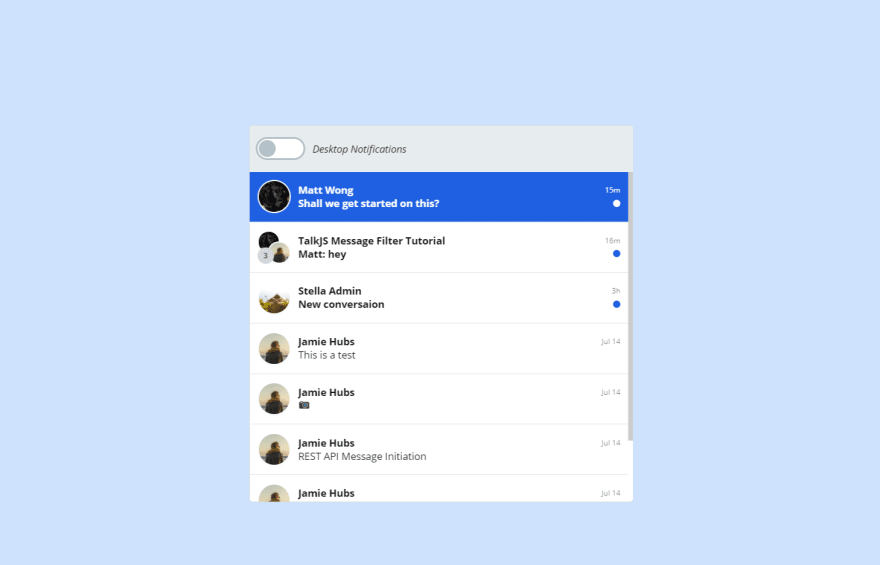
Note: Other than plain JavaScript, TalkJs SDK supports Vue, Angular, and React. And the API will be the same across these frameworks. You can check out our docs for more information.
Listening for Unread Conversation Changes
Now that our Inbox is set up, we can start listening for any unread conversations. This is made easy by the TalkJS Session.unreads object.
The “On” method of the Session.unreads object fires a “change” event right after TalkJS loads, and every time the amount of unread conversations changes. The event handler gets an array of objects with information about the unread conversations.
window.talkSession.unreads.on("change", function (unreadConversations) {
console.log(unreadConversations)
});
The _unreadConversations_is a JSON array that looks something like this:
[
{
"lastMessage": {
"conversation": {
"id": "unread1",
"custom": {
"category": "test123"
},
"topicId": null,
"subject": "TalkJS Unread Message Counter tutorial",
"welcomeMessages": null,
"photoUrl": null
},
"isByMe": false,
"senderId": "135487",
"sender": {
"id": "135487",
"name": "Stella Admin",
"welcomeMessage": "Hey there! How are you? :-)",
"photoUrl": "https://picsum.photos/200",
"role": "Admin",
"configuration": "Admin",
"custom": {},
"availabilityText": null,
"locale": null,
"email": "<suppressed>",
"phone": "<suppressed>"
},
"body": "New conversation",
"type": "text",
"timestamp": 1627806520159,
"read": false,
"origin": "web",
"custom": {
"textMessage": "true"
},
"attachment": null,
"location": null
}
}
]
Rendering the Unread Counter
Now that we have figured out how to get the data on unread conversations of a user, we can use the data to display information to the user however we want!
Let me show an example of this using a simple HTML span tag and JavaScript to update the unread conversations counter.
Define a heading that contains the placeholder for the unread counter in a span tag.
<div>
<h1>You have <span id="unreadCount" class="bold_blue"> 0 </span> unread conversations.</h1>
</div>
Define an event handler for the Session.unreads.on() method that updates the unread counter whenever the TalkJS app loads or the unread conversation data changes. We can check the length of the array provided to the Handler to determine the number of unread conversations.
window.talkSession.unreads.on("change", function (unreadConversations) {
var amountOfUnreads = unreadConversations.length;
document.getElementById("unreadCount").textContent= amountOfUnreads;
});
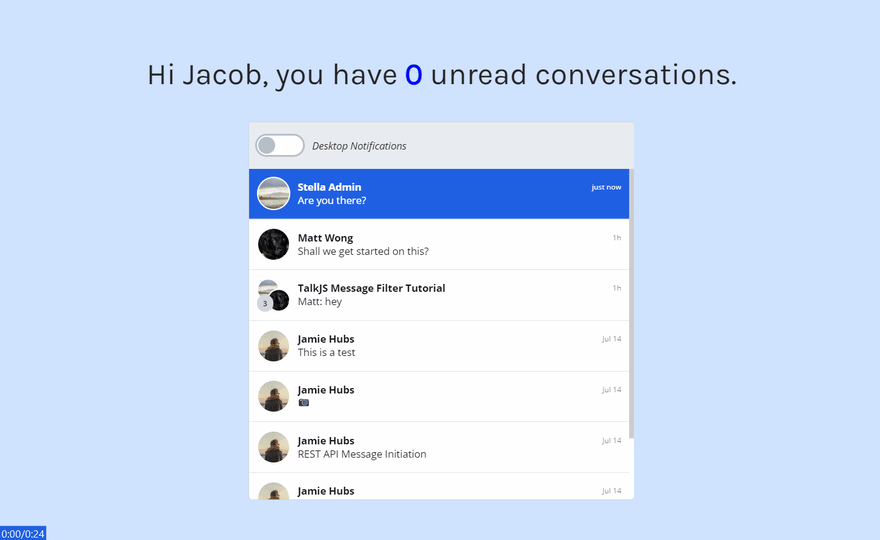
That’s it! This was a simple example, but as you have plenty of information available for an unread conversation, you can get creative and implement better UX-friendly ways to display the counter!
If you run into any issues, please get in touch via the popup on our website or send an email to dev@talkjs.com.