This tutorial will teach us how to add quick replies to a chat that was built with TalkJS. We'll do it by using HTML Panels.
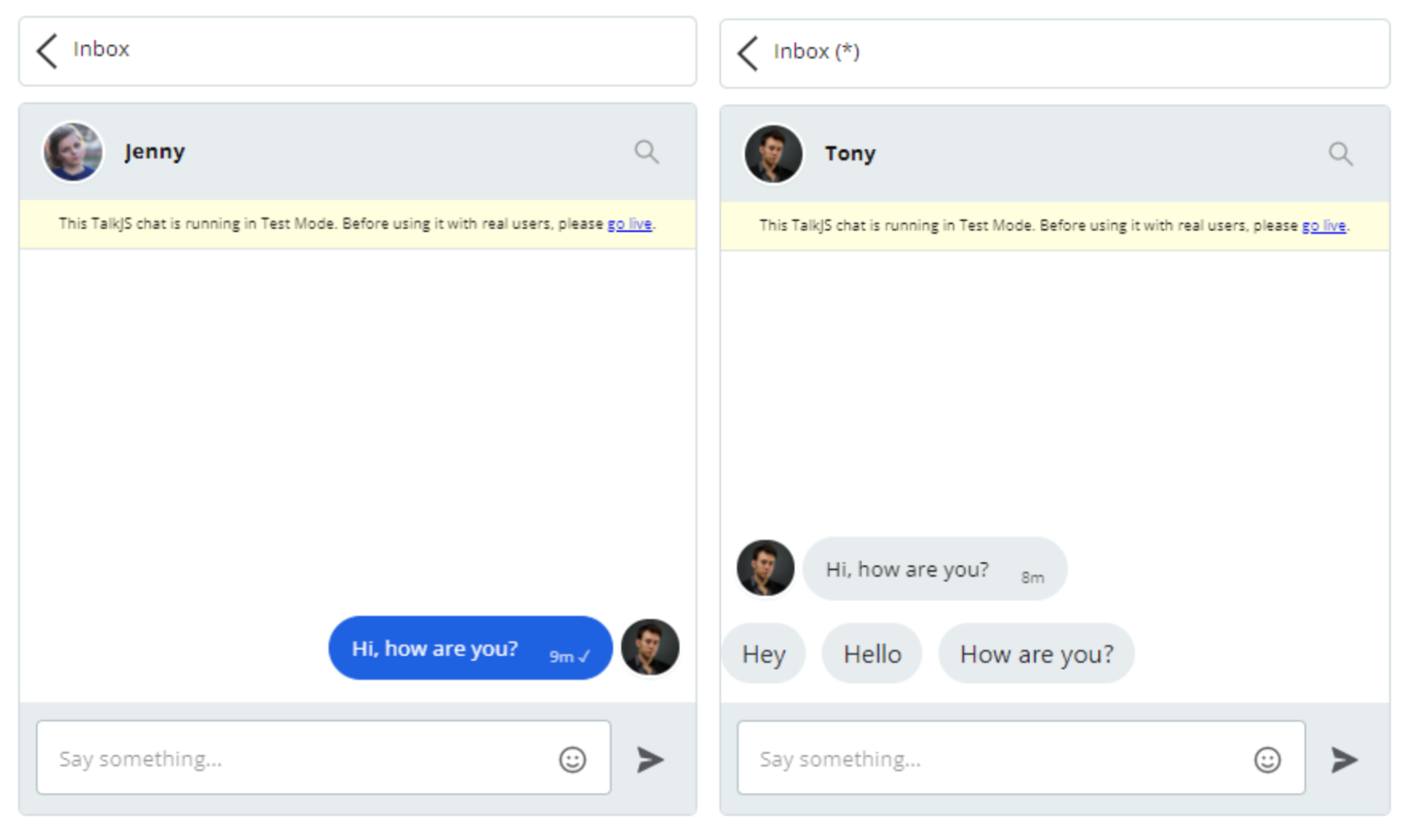
Setting Up a Session and Creating an Inbox.
A TalkJS Session is a connection between a User and a TalkJS Account, except that the users don’t directly log in to the TalkJS account.
Below, we create a user and bind that user to a TalkJS Inbox instance using the Session. Let’s define a TalkJS Session using the new Talk.Session(options) constructor and pass our APP_ID and the User object.
var me = new Talk.User({
id: '0123456',
name: 'Jenny',
email: 'jenny@demo.com',
photoUrl: 'https://randomuser.me/api/portraits/women/72.jpg',
});
window.talkSession = new Talk.Session({
appId: "APP_ID",
me: me,
});
Once the session and user are created, Let’s make a TalkJS Inbox to chat with other TalkJS Users.
var conversationBuilder = talkSession.getOrCreateConversation(
Talk.oneOnOneId(me, other)
);
conversationBuilder.setParticipant(me);
conversationBuilder.setParticipant(other);
var inbox = talkSession.createInbox({ selected: conversationBuilder });
inbox.mount(document.getElementById('talkjs-container'));
What Is HtmlPanel?
HtmlPanel in TalkJS Chat SDK allows you to extend the chat interface by adding HTML components like buttons, calendars, and hyperlinks, et cetera, in an iframe in your chats. HtmlPanel should only be created using the createHtmlPanel method through Inbox, Chatbox, or Popups.
Implementing Quick Replies using HtmlPanel and Buttons
Here, we will be using inbox.createHtmlPanel
method and put a custom HTML just above the message field. The custom HTML contains a set of buttons with labels that act as quick replies for the user.
The quick_replies.html
document contains the code component that will be placed into the iframe of the inbox. The below div tag can have hardcoded buttons with the quick reply text labels or a more practical approach is to add them dynamically based on the list from the javascript.
quick_replies.html:
<body>
<div id="btn-container"></div>
</body>
The document method getElementById()
returns the DOM element of the specified ID and once the element is retrieved, we loop through the count of the length of the messageOptions array and create buttons for each quick reply message using the createElement()
method. Once the button is configured, we append the buttons to the DOM element using the appendChild()
method.
script.js:
var messageOptions = ["Hey", "Hello", "How are you?"]; // Message list.
inbox
.createHtmlPanel({
url: 'quick_replies.html', // or the absolute path
height: 50,
show: true,
})
.then(function (htmlPanel) {
htmlPanel.DOMContentLoadedPromise.then(function () {
// ... do work that includes manipulation of the DOM.
const buttonsContainer = htmlPanel.window.document.getElementById("btn-container");
for (let i = 0; i < messageOptions.length; i++){
const button = document.createElement("button");
button.className = "btn button";
button.innerText = messageOptions[i];
button.addEventListener("click", () => {
conversationBuilder.sendMessage(messageOptions[i]);
htmlPanel.hide();
});
buttonsContainer.appendChild(button);
}
});
});
The createHtmlPanel
method comprises three options. URL of the HTML component, Height of the panel, and whether to show it or not.
Once the HtmlPanel is ready, it exposes the DOMContentLoadedPromise. We can register the event listeners in the DOMContentLoadedPromise to invoke ConversationBuilder’s sendMessage() method.
Once the message is sent, we can hide the quick reply options by setting the visibility of the HtmlPanel using the htmlPanel.hide()
method.
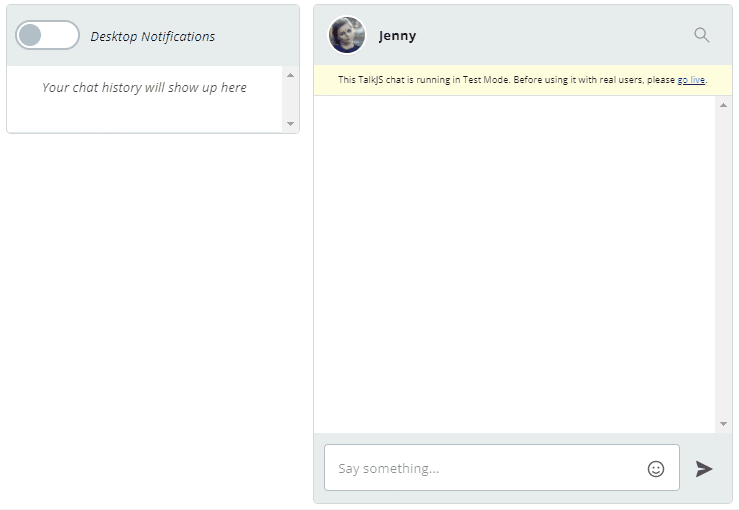
That’s it! This was a simple example, but as the HTMLpanels are very flexible, you can get creative and come up with your own version of quick replies for your TalkJS Chat implementation. You can also explore more at TalkJS HtmlPanel.
If you run into any issues, please get in touch via the popup on our website or send an email to dev@talkjs.com.