In this tutorial, will show you how to send announcements and broadcast messages to multiple conversations in a TalkJS inbox. We will use the JavaScript Chat SDK along with the TalkJS System Messages API to implement the announcements.
Why send announcements?
Before we go any further, let us understand why one might implement announcements in their application’s chat from the following use case examples:
- A back-end admin is sending organizational updates to all team channels.
- Sending Chat usage policy or privacy policy notifications to all active conversations.
- General notifications for all users that are currently engaged in a TalkJS conversation.
Getting started with TalkJS Conversations
TalkJS Conversations allow two or more people to communicate in a group chat. To get started, simply fork and clone the https://github.com/talkjs/talkjs-examples repository in your local dev machine and start working on the index.html located at “talkjs-examples\howtos\how-to-create-a-channel-list-in-talkjs-chat”.
You may have to replace the APP_ID string with your actual TalkJS application ID.
const session = new Talk.Session({
appId: "APP_ID",
me: me
});
Note: You can refer to the guide on setting up a conversation list using TalkJS to get more details on the how-to-create-a-channel-list-in-talkjs-chat Github repository.
You should see a list of test conversations when you open “index.html” in a web browser.
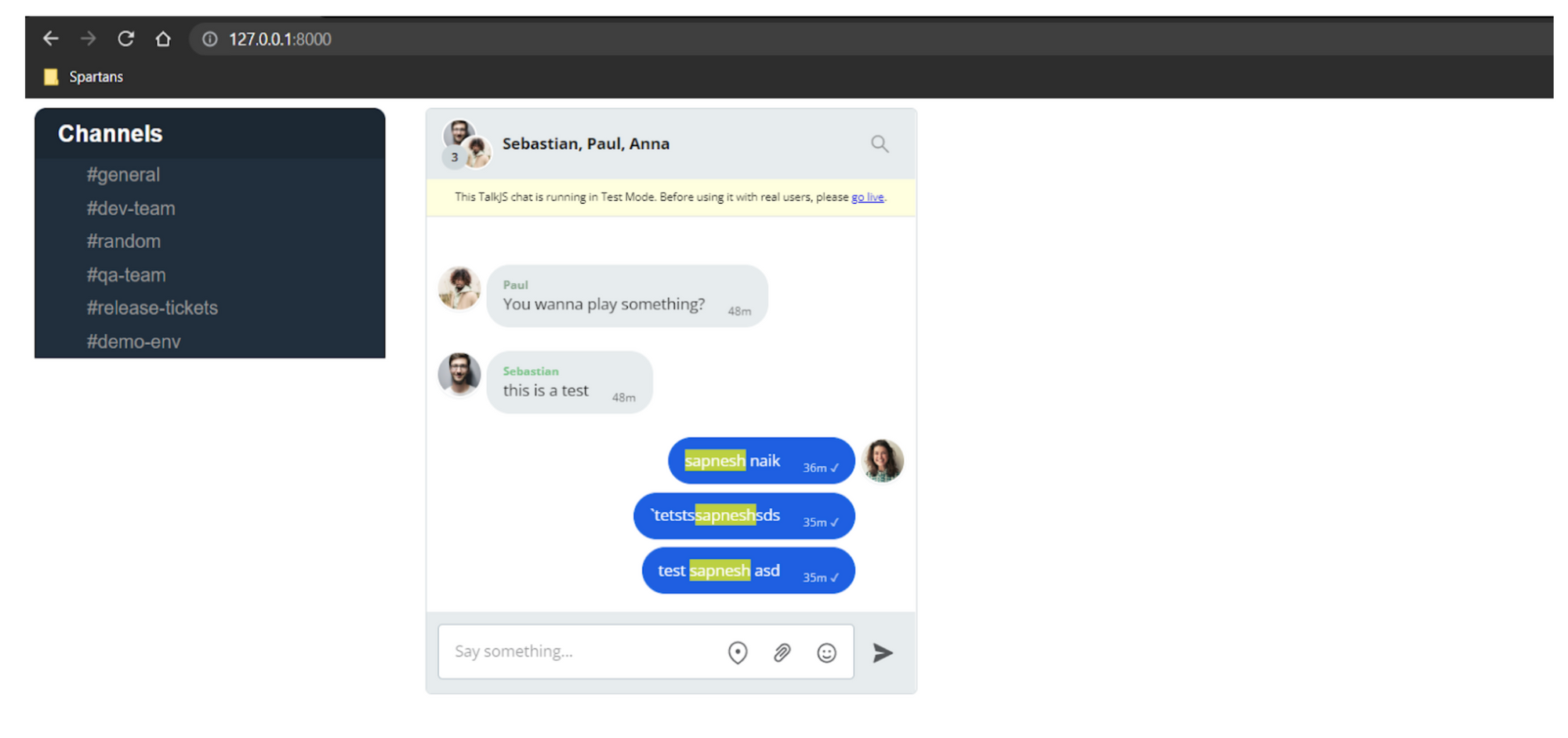
In the next sections, we will build on top of this setup to implement channel-wide announcements.
Building a new HTML form to send announcements
In a typical scenario, announcements will be sent from a backend team and would ideally be done from a separate page altogether. We will create an example admin.html page with a simple HTML form that takes an input for the announcement text and a button to send the announcements.
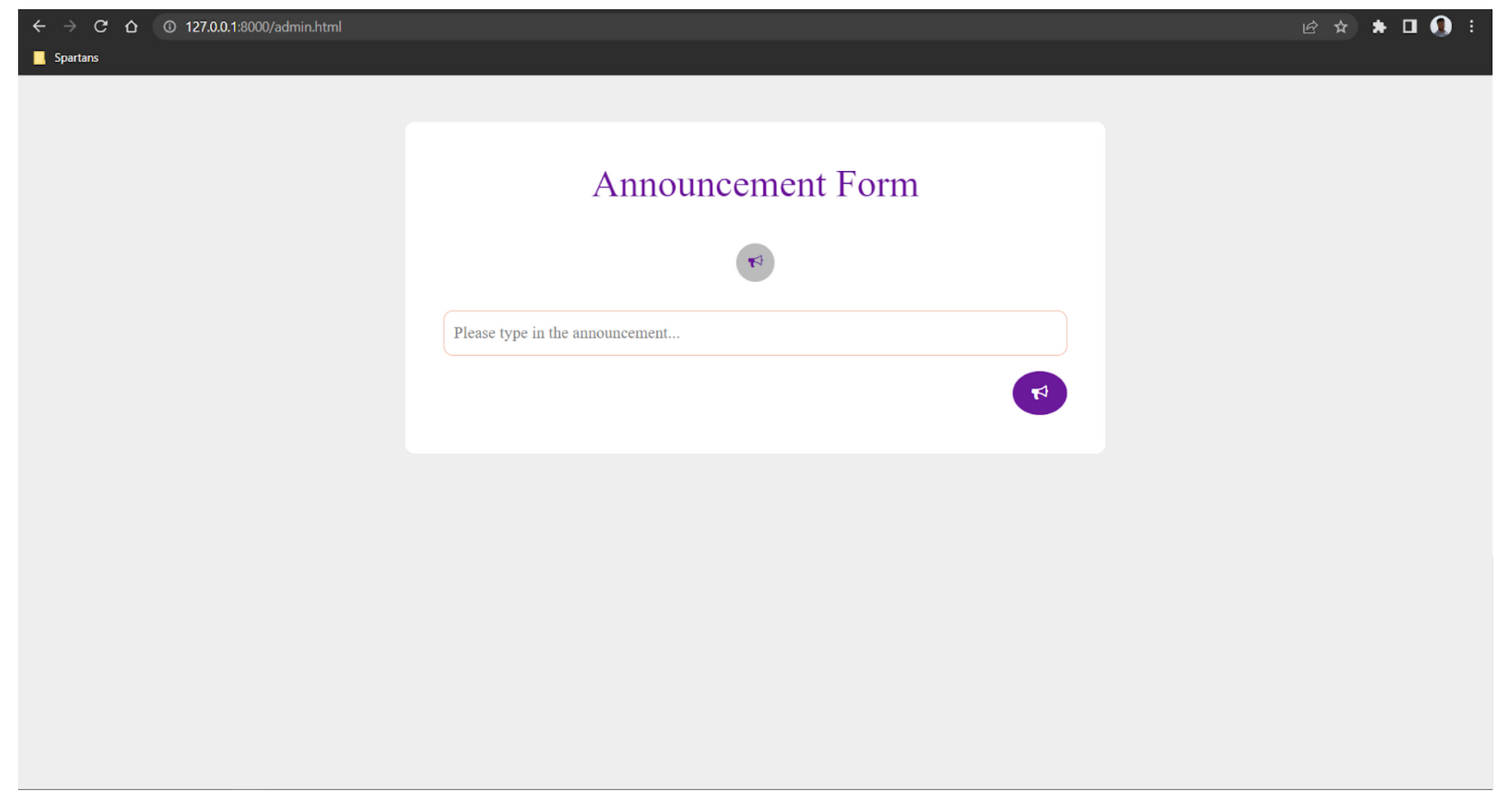
Here is the sample code for the HTML form.
<form id="regForm">
<h1 id="register">Announcement Form</h1>
<div class="all-steps" id="all-steps"> <span class="step"><i class="fa fa-bullhorn"></i></div>
<div class="tab">
<p> <input placeholder="Please type in the announcement..." oninput="this.className = ''" name="fname"></p>
</div>
<div style="overflow:auto;" id="nextprevious">
<div style="float:right;"> <button type="button" id="prevBtn"</button> </div>
</div>
</form>
Sending announcements
Now, we can handle the OnClick event of the HTML button from JavaScript to fetch the input text and send it out as a System Message across all conversations.
function butttonOnClickHander() {
announceText = document.getElementById("announcement").value
console.log(announceText)
}
Step 1: Get all or a filtered list of conversations
We can use the TalkJS REST API to get a list of all conversations so that we can send a system message across them all.
If you want to send announcements to only specific conversations then you can also use the filtering capabilities of our Conversations API. Currently, you can filter conversations based on custom fields and users. For more information, see our REST API documentation.
Note: Please replace the “secret-key” and “APP_ID” with your own secret key and APP_ID obtained from the TalkJS dashboard.
function getAllConversations() {
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Authorization", "Bearer secret-key");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'follow'
};
return fetch("https://api.talkjs.com/v1/APP_ID/conversations", requestOptions)
.then(response => response.text())
.then(function(response) {
conversationIDs = []
for (let conversation of response.data) {
conversationIDs.push(conversation.id)
}
return conversationIDs
})
.catch(error => console.log('error', error));
}
Step 2: Send the announcement to all Conversations.
Now, we can iterate over all the conversationIDs to send the announcement across all channels.
function sendAnnouncement(conversationID, announceText) {
console.log("here")
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Authorization", "Bearer secret-key");
var raw = JSON.stringify([
{
"text": announceText,
"type": "SystemMessage"
}
]);
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.talkjs.com/v1/APP_ID/conversations/"+conversationID+"/messages", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
}
Let’s combine all our steps into the button onClick handler and send out the announcements.
function butttonOnClickHander() {
announceText = document.getElementById("announcement").value
getAllConversations().then((result) => {
console.log(result)
for (let conversationID of result) {
sendAnnouncement(conversationID, announceText)
}
})
}
The final solution looks something like this.
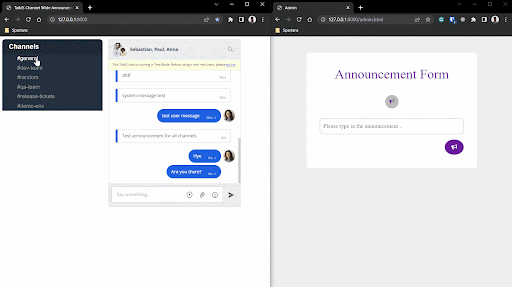
If you run into any issues, please get in touch via the popup on our website or send an email to dev@talkjs.com.