Mentions are a powerful feature of any chat. They allow users to cut through the noise and alert each other to topics that really demand their attention. In TalkJS, you can enable user mentions for your chat directly from your dashboard.
In high-volume chats, you may want to make messages with mentions yet easier to find. For example, by adding a tab or a toggle button that shows the user only those conversations or messages in which they are mentioned.
This guide shows you how to add a toggle button to your chat to filter messages that @-mention the current user.
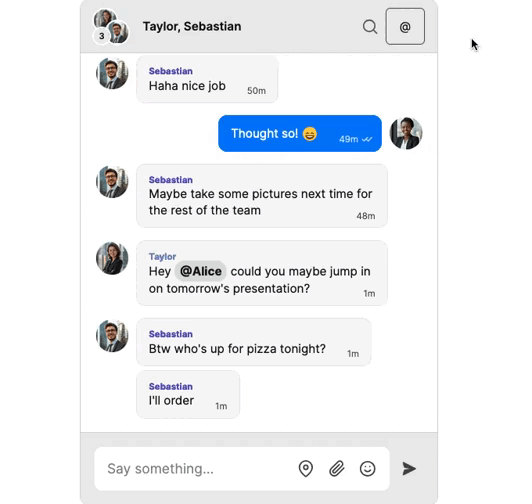
Note: Code snippets in this guide use the JavaScript SDK with the chatbox pre-built chat UI, but can be adjusted to work with the inbox or popup UI, as well as with the React, React Native, or Flutter SDKs.
1. Create a mentions toggle button
Begin by adding a toggle button to your chat that allows the user to easily show their mentions, or switch back to all messages. As with any theme customization, you can add such a toggle button to your chat using the theme editor.
With the following code snippet, you can add an action button for the toggle, which you can add to the ChatHeader component of your theme:
<!-- Set the filter toggle field and toggle-enabled state -->
<t:set toggleField="filterToggle-{{conversation.me.id}}" />
<t:set toggleEnabled="{{conversation.custom[toggleField] == 'true'}}" />
<!-- Render a mentions button to toggle the mentions filter -->
<ActionButton
action="toggleFilter"
class="action-button {{toggleEnabled | then: 'toggle-enabled' | else: ''}}"
data-currentState='{{toggleEnabled}}'
data-toggleField="{{toggleField}}"
data-custom="{{conversation.custom}}">
<!-- Use the '@' symbol as button text for the filter toggle -->
@
</ActionButton>
To style the toggle button, you can add any styling to the ChatHeader
component's <style>
tag. For example, to make the button dark when toggled, you could use the following:
.action-button { /* Your toggle button's default style */ }
.action-button.toggle-enabled[data-action] {
color: white;
background-color: black;
}
This gives you something like the following for the button’s active and inactive state:
The button doesn’t do anything yet. You can change that, by creating a mentions filter that can be turned on or off when your user clicks the toggle button.
2. Add a mentions filter
Create a filter that filters for all and only those messages that contain an @-mention of the current user.
Identify messages that mention a user
For a mentions filter to work, it must be able to identify which messages mention a user. TalkJS doesn’t include a dedicated message field for storing mentions data out of the box, but you can use custom message fields for this purpose, for example with the following code:
// Regular expression to find "@username" mentions
let mentionRegex = /@(\w+)/g;
// Handle message send event
chatbox.onSendMessage((e) => {
// Process only if the message contains text
if (e.message.text) {
let customFields = {};
// Extract all mentions from the message text
let matches = e.message.text.matchAll(mentionRegex);
// Create a custom field for each mention
for (let match of matches) {
customFields[`mentions-${match[1]}`] = "true";
}
// Add custom fields to the message data
e.override({ custom: customFields });
}
});
For each text-based message—as opposed to, for example, images or location shares—this code checks whether a user is mentioned, and sets that information to a custom message field. For example, if a message mentions Alice, then this filter will set the following custom data on that message:
"custom": {
"mentions-Alice": "true",
}
This way, you can identify all messages that mention a user.
Apply or remove the mentions filter when a user clicks the toggle button
To make your toggle button work, you can now add a custom message action handler to your JavaScript code that either applies or removes a mentions filter whenever the user clicks the toggle button. For example, you could use the following:
// Filter to show only messages that mention the current user
const mentionsPredicate = { custom: {[`mentions-${me.name}`]: ["==", "true"] } };
// Keep the toggle's state in localStorage and, if applicable, apply the mentions filter
if(localStorage.getItem("filterMentions") == "true") {
chatbox.setMessageFilter(mentionsPredicate);
}
// Handle the custom action that's triggered when the toggle button is clicked
chatbox.onCustomConversationAction((action) => {
// Update a custom conversation field to keep the toggle state,
// and make it available in the theme
conversation.setAttributes({
custom: {
[`filterToggle-${session.me.id}`]: action.params.currentState ? "false" : "true"
}
});
// Sync the custom conversation data with the server
chatbox.select(conversation);
// Update localStorage to keep the setting in sync
localStorage.setItem("filterMentions", (!action.params.currentState).toString());
// Set the message filter based on the current state
// If the toggle is currently off, set the filter
if (!action.params.currentState) {
chatbox.setMessageFilter({
custom: {mentionsPredicate}
});
// If the toggle is currently on, set no filter
} else {
chatbox.setMessageFilter(null);
}
});
This code snippet creates a filter that only shows messages that mention the current user. It keeps the mention button’s current state in localStorage
, to be able to know this state at page load time. In addition, it sets a custom field on the conversation to keep track of the toggle state, and uses setMessageFilter on the chatbox to apply the mentions filter.
If the user currently has the mentions toggle turned off, then clicking the button turns the mentions filter on. In this case, the chat only shows messages that mention the current user. If the user currently has the mentions toggle turned on, then clicking the button turns the filter off, and the chat will show all messages, regardless of whether they mention the current user.
The result will be something like the following, where activating the mentions toggle shows only messages that mention the current user:
You now have a working mentions toggle button in your chat, that allows users to filter their messages based on whether the message mentions them.
Do you have questions about filtering user mentions? We’re happy to help. Get in touch.