TalkJS is a highly customizable chat API with a pre-built UI that helps teams deliver a rich chat experience in hours instead of months. TalkJS also makes it possible to personalize chat experiences on your websites and mobile products with custom templates using a feature called HTML panels.
In this article, we will explore how to create a 'Get notified by email' form with HTML panels in TalkJS.
Setting up a TalkJS UI
Create an account on TalkJS and retrieve your App_Id
, this would be used to connect your App to the TalkJS API.
To set up a Chat UI, we need to install the TalkJS SDK for our preferred framework. We would be using Vue.js for this article.
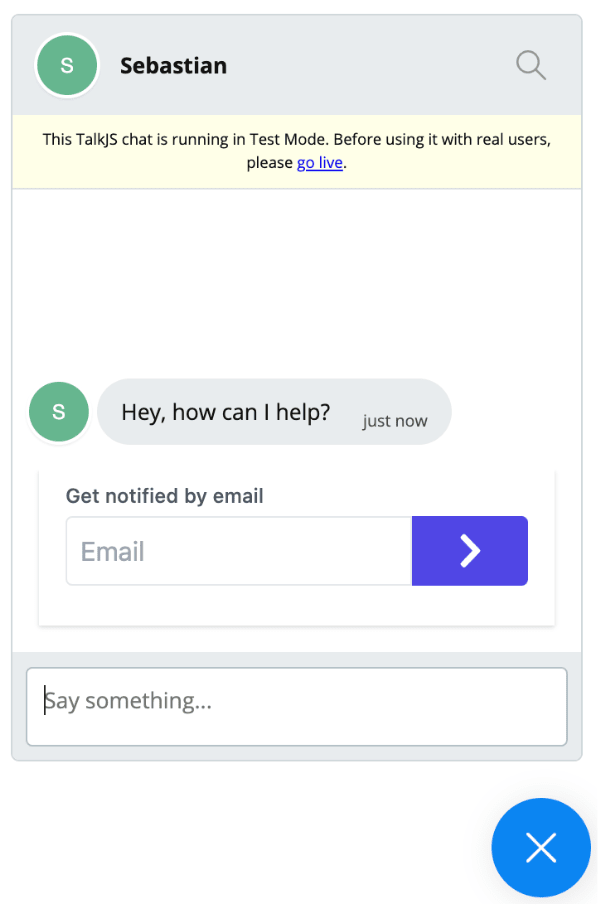
Please refer to the official documentation on how to install and set up the TalkJS SDK for Vue.
If fully set up, import TalkJs into your app and add a container element to render the Chat UI like so:
<! ../components/HtmlPanel.vue ->
<template>
<div class="talkjs-container"></div>
</template>
<script>
import Talk from 'talkjs';
</script>
Next, we need to connect our application with TalkJS and create a user session. This would be done in the mounted hook as seen in the snippet below:
// ../components/HtmlPanel.vue
...
mounted: function () {
Talk.ready.then(async function () {
const me = new Talk.User({
id: parseInt(Math.random() * 500000).toString(),
name: "Alice",
email: "demo@talkjs.com",
welcomeMessage: "Hey there! How are you? :-)",
role: "default",
});
const session = new Talk.Session({
appId: "YOUR_APP_ID",
me: me,
});
...
Remember to replace YOUR_APP_ID
with the appId found in the TalkJS dashboard.
After creating a user session, we’ll create another user which would help us simulate a conversation in our chat UI and then mount our chat UI like so:
// ../components/HtmlPanel.vue
...
const other = new Talk.User({
id: parseInt(Math.random() * 500000).toString(),
name: "Sebastian",
email: "demo@talkjs.com",
welcomeMessage: "Hey, how can I help?",
role: "default",
});
const conversation = session.getOrCreateConversation(
Talk.oneOnOneId(me, other)
);
conversation.setParticipant(me);
conversation.setParticipant(other);
const popup = session.createPopup(conversation, {
keepOpen: true,
});
await popup.mount({ show: true });
...
Please note these are hardcoded users and in actual sense you would need to implement the logic to retrieve users from your application’s backend. To know more about conversations in TalkJS, you can check out the official documentation.
If all goes well, you should see the TalkJS UI when you visit your application.
Creating the form
The TalkJS Chat UI is rendered inside an Iframe which means you can’t manipulate the DOM directly. This is where HTML panels come in, it helps us insert a custom UI with functionality inside the TalkJS UI.
To build our custom UI, we’ll create an HTML template (styled to your taste) with the snippet below:
<! ../public/static/get-notified.html-->
<form id="form-container">
<h3>Leave your email address</h3>
<input type="text" name="email" id="email" placeholder="Email">
<button id="submit" type="submit">Submit</button>
</form>
This should be placed in the public directory of your vue project so as to help webpack serve it a static file.
When that is done, we can now wrap our form inside a HTML panel like so:
// ../components/HtmlPanel.vue
...
Talk.ready.then(async function() {
...
const htmlPanel = await popup.createHtmlPanel({
url: "./static/get-notified.html",
height: 150,
show: true,
})
})
Handling form Submissions
Finally, you can handle form submission right from the Chat UI by listening and responding to events triggered from the HTML Panel.
To submit a form, add an event listener to the submit button and handle your request like so:
Talk.ready.then(async function() {
...
const button = htmlPanel.window.document.getElementById("submit")
button.addEventListener("click", () => {
// handle form submission
alert("submission successful");
htmlPanel.hide();
});
})
Conclusion
TalkJs is a powerful tool that can help boost your product engagement, it also provides varying functionalities that can help you achieve this. To know more, you can checkout the official website.